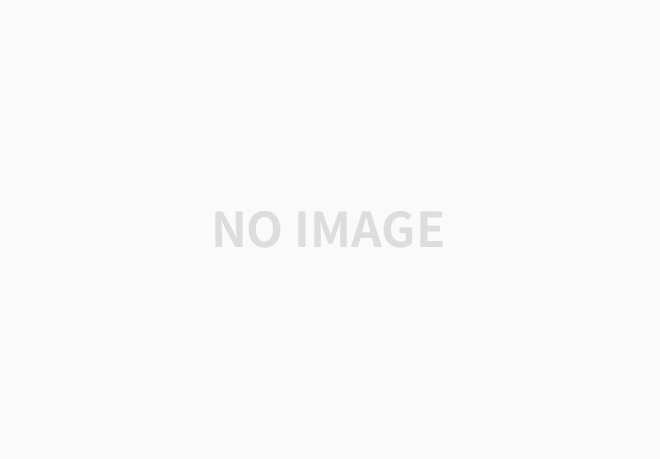
현재 테스트 광고 단위를 사용하는데
배너의 크기를 조절하던 중 오류가 났다.
분명 커스터마이징이 가능한 줄 알았는데 애드몹에서 지정된 사이즈로만
변경 할 수 있던 것이다.
본 프로젝트에는 크기에 맞춤을 적용하였다.
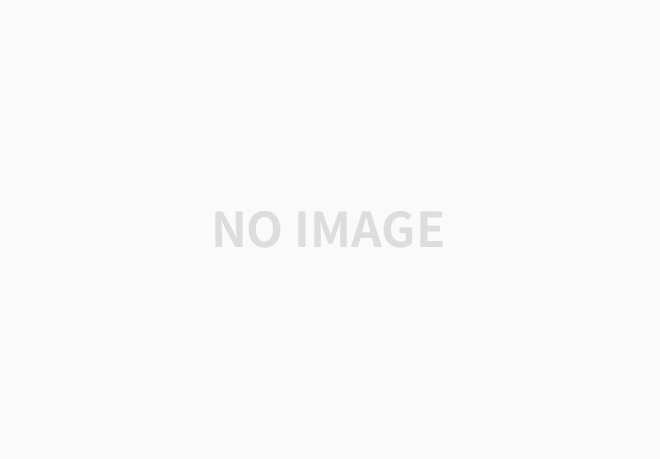
https://developers.google.com/admob/unity/banner?hl=ko
배너 광고 | Unity | Google for Developers
이 페이지는 Cloud Translation API를 통해 번역되었습니다. 배너 광고 컬렉션을 사용해 정리하기 내 환경설정을 기준으로 콘텐츠를 저장하고 분류하세요. 배너 보기는 화면 안의 한 지점을 차지하는
developers.google.com
using UnityEngine;
using GoogleMobileAds.Api;
using System;
using System.Drawing;
public class BannerManager : MonoBehaviour
{
public static BannerManager instance;
public static BannerManager Instance
{
get
{
// Singleton 패턴을 이용하여 단일 인스턴스 반환
if (instance == null)
{
instance = FindObjectOfType<BannerManager>();
if (instance == null)
{
GameObject adMobManagerGO = new GameObject();
instance = adMobManagerGO.AddComponent<BannerManager>();
adMobManagerGO.name = "AdMobManager";
DontDestroyOnLoad(adMobManagerGO);
}
}
return instance;
}
}
public string _adUnitId;
// 광고 뷰 객체
BannerView _bannerView;
public void Awake()
{
// 싱글톤 인스턴스 설정
if (instance == null)
{
instance = this as BannerManager;
DontDestroyOnLoad(gameObject);
}
else
{
Destroy(gameObject);
}
}
public void Start()
{
//// Google Mobile Ads SDK 초기화
MobileAds.Initialize((InitializationStatus initStatus) =>
{
// SDK 초기화 콜백
Debug.Log("Google Mobile Ads SDK initialized.");
// 광고 뷰 생성
//_bannerView = new BannerView(_adUnitId, AdSize.Banner, AdPosition.Bottom);
//AdSize adSize = new AdSize(350, 50);
//_bannerView = new BannerView(_adUnitId, adSize, AdPosition.Bottom);
CreateBannerView();
ListenToAdEvents();
});
//CreateBannerView();
#if UNITY_ANDROID
_adUnitId = "ca-app-pub-3940256099942544/6300978111"; // 안드로이드 테스트 광고 단위 ID
#elif UNITY_IPHONE
_adUnitId = "ca-app-pub-3940256099942544/2934735716"; // iOS 테스트 광고 단위 ID
#else
_adUnitId = "unused"; // 기타 플랫폼 또는 테스트에 사용되지 않는 경우
#endif
}
public void CreateBannerView()
{
Debug.Log("Creating banner view");
// 기존 배너 뷰가 있으면 제거
//if (_bannerView != null)
//{
// _bannerView.Destroy();
//}
// Use the AdSize argument to set a custom size for the ad.
AdSize adSize = AdSize.GetCurrentOrientationAnchoredAdaptiveBannerAdSizeWithWidth(AdSize.FullWidth);
//_bannerView = new BannerView(_adUnitId, adSize, AdPosition.Bottom);
_bannerView = new BannerView(_adUnitId, adSize, AdPosition.Bottom);
ListenToAdEvents();
}/// <summary>
/// Creates the banner view and loads a banner ad.
/// </summary>
public void LoadAd()
{
// create an instance of a banner view first.
if (_bannerView == null)
{
CreateBannerView();
}
// create our request used to load the ad.
var adRequest = new AdRequest();
// send the request to load the ad.
Debug.Log("Loading banner ad.");
_bannerView.LoadAd(adRequest);
}
/// <summary>
/// listen to events the banner view may raise.
/// </summary>
public void ListenToAdEvents()
{
// Raised when an ad is loaded into the banner view.
_bannerView.OnBannerAdLoaded += () =>
{
Debug.Log("Banner view loaded an ad with response : "
+ _bannerView.GetResponseInfo());
};
// Raised when an ad fails to load into the banner view.
_bannerView.OnBannerAdLoadFailed += (LoadAdError error) =>
{
Debug.LogError("Banner view failed to load an ad with error : "
+ error);
};
// Raised when the ad is estimated to have earned money.
_bannerView.OnAdPaid += (AdValue adValue) =>
{
Debug.Log(String.Format("Banner view paid {0} {1}.",
adValue.Value,
adValue.CurrencyCode));
};
// Raised when an impression is recorded for an ad.
_bannerView.OnAdImpressionRecorded += () =>
{
Debug.Log("Banner view recorded an impression.");
};
// Raised when a click is recorded for an ad.
_bannerView.OnAdClicked += () =>
{
Debug.Log("Banner view was clicked.");
};
// Raised when an ad opened full screen content.
_bannerView.OnAdFullScreenContentOpened += () =>
{
Debug.Log("Banner view full screen content opened.");
};
// Raised when the ad closed full screen content.
_bannerView.OnAdFullScreenContentClosed += () =>
{
Debug.Log("Banner view full screen content closed.");
};
}/// <summary>
/// Destroys the banner view.
/// </summary>
public void DestroyAd()
{
if (_bannerView != null)
{
Debug.Log("Destroying banner view.");
_bannerView.Destroy();
_bannerView = null;
}
}
}
using UnityEngine;
using Firebase.Analytics;
public class AdManager : MonoBehaviour
{
private static AdManager instance;
public static AdManager Instance
{
get
{
if (instance == null)
{
instance = FindObjectOfType<AdManager>();
if (instance == null)
{
GameObject adManagerGO = new GameObject();
instance = adManagerGO.AddComponent<AdManager>();
adManagerGO.name = "AdManager";
DontDestroyOnLoad(adManagerGO);
}
}
return instance;
}
}
private void Awake()
{
if (instance == null)
{
instance = this as AdManager;
DontDestroyOnLoad(gameObject);
}
else
{
Destroy(gameObject);
}
}
public void Start()
{
// Initialize Firebase Analytics
FirebaseAnalytics.SetAnalyticsCollectionEnabled(true);
FirebaseAnalytics.LogEvent(FirebaseAnalytics.EventAppOpen);
BannerManager.Instance.LoadAd();
}
public void ShowInterstitialAd()
{
// Show interstitial ad logic here
// For example, assuming _interstitialAd is an instance of your interstitial ad
// and it's shown successfully, log the event
LogInterstitialAdShownEvent();
}
private void LogInterstitialAdShownEvent()
{
// Log an event indicating the interstitial ad was shown
FirebaseAnalytics.LogEvent("interstitial_ad_shown");
}
}
'산대특' 카테고리의 다른 글
플레이콘솔 리더보드에 점수 올리기 (0) | 2024.06.26 |
---|---|
2D 3Matchpuzzle - 매치 시 파괴 및 로드 + 힌트 (0) | 2024.05.20 |
2D 3Matchpuzzle - 블록 위치 찾기 테스트 (0) | 2024.05.16 |
2D 3Matchpuzzle - 빈공간 찾기 (0) | 2024.05.14 |
Download Python (0) | 2024.05.08 |