블록에 BoxCollider2D를 부착한 후 클릭을 하면
해당 블록의 배열로서의 위치와 Vector2의 위치가 출력됨
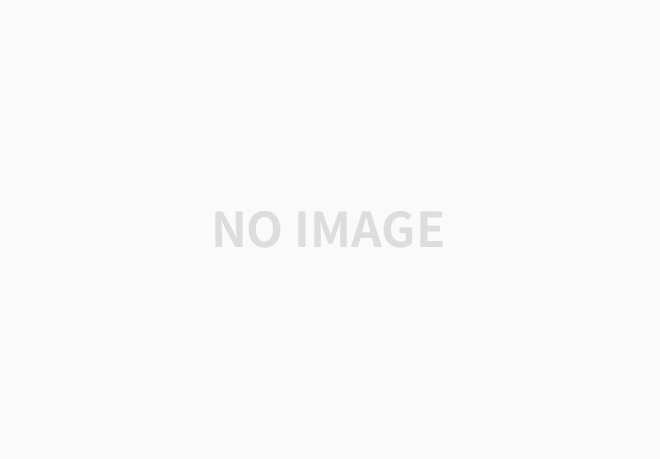
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.U2D;
public class AtlasManager : MonoBehaviour
{
public static AtlasManager instance;
public SpriteAtlas blockAtlas;
//싱글톤
private void Awake()
{
//AtlasManager 클래스의 인스턴스를 instance에 할당
instance = this;
}
}
using System;
using System.Collections;
using System.Collections.Generic;
using System.Text;
using UnityEngine;
using UnityEngine.U2D;
using Random = UnityEngine.Random;
public class Test : MonoBehaviour
{
public Board board;
private void Start()
{
board.CreateBoard();
}
private void Update()
{
if (Input.GetMouseButtonDown(0))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
Debug.DrawRay(ray.origin, ray.direction * 100f, Color.red, 1);
RaycastHit2D raycastHit2D = Physics2D.Raycast(ray.origin, ray.direction);
if (raycastHit2D.collider != null)
{
Block block = raycastHit2D.collider.GetComponent<Block>();
Debug.Log($"[{block.row}, {block.col}] ({block.transform.position.x}, {block.transform.position.y}), {block.blockType}");
}
}
}
}
using System;
using System.Collections;
using System.Collections.Generic;
using System.Text;
using UnityEngine;
using Random = UnityEngine.Random;
public class Board : MonoBehaviour
{
private Block[,] board; //1차원 배열이 Block들을 관리
public GameObject blockPrefab;
public void CreateBoard()
{
//크기가 9인 BlockType의 1차원 배열 만들기
board = new Block[5, 9]; // 2차원 배열로 변경
//5행 9열의 Block타입의 2차원 배열 만들기
for (int i = 0; i < board.GetLength(0); i++)
{
for (int j = 0; j < board.GetLength(1); j++)
{
CreateBlock(i, j);
}
}
PrintBoard();
}
public void CreateBlock(int row, int col)
{
Vector2 pos = new Vector2(col, row);
Block.BlockType blockType = (Block.BlockType)Random.Range(0, 5);
GameObject blockGo = Instantiate(blockPrefab);
Block block = blockGo.GetComponent<Block>();
block.Init(blockType);
block.SetPosition(pos);
//배열의 요소에 블록 넣기
board[row, col] = block;
}
public void PrintBoard()
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < board.GetLength(0); i++)
{
for (int j = 0; j < board.GetLength(1); j++)
{
sb.Append($"({i},{j})"); // StringBuilder에 문자열 추가
}
sb.AppendLine(); // 새로운 행 추가
}
Debug.Log(sb); // StringBuilder에 저장된 문자열을 출력
}
}
using System.Collections;
using System.Collections.Generic;
using TMPro;
using Unity.Mathematics;
using UnityEngine;
public class Block : MonoBehaviour
{
public enum BlockType
{
Blue, Gray, Green, Pink, Yellow
}
public BlockType blockType;
public SpriteRenderer spriteRenderer;
public TMP_Text debugText;
public int row;
public int col;
public void Init(BlockType blockType)
{
this.blockType = blockType;
//이미지 변경
ChangeSprite(blockType);
}
public void ChangeSprite(BlockType blockType)
{
//블록의 이름을 넣어서 아틀라스에서 같은 이름인 sprite를 찾고 할당
Sprite sp =
AtlasManager.instance.blockAtlas.GetSprite(blockType.ToString());
spriteRenderer.sprite = sp;
}
public void SetPosition(Vector2 pos)
{
transform.position = pos;
var index = Position2Index(pos);
row = index.row;
col = index.col;
debugText.text = $"[{index.row}, {index.col}]";
}
public static (int row, int col) Position2Index(Vector2 pos)
{
return ((int)pos.y, (int)pos.x);
}
public static (int x, int y) Index2Position(Vector2 index)
{
return ((int)index.x, (int)index.y);
}
}
'Study > Unity' 카테고리의 다른 글
[Unity] 클릭된 객체들의 정보를 인포로 저장하기 (1) | 2024.06.11 |
---|---|
[Unity] 팝업 스킬 중복 제거 및 셔플 시 중복 제거 (1) | 2024.06.10 |
[Unity] 클릭시 색상 변경 및 Select버튼 On/Off (0) | 2024.06.09 |
[Unity] Random Skill Button & Select Button (0) | 2024.06.08 |
[Unity] 클릭시 json에 있는 파일들 랜덤으로 가져오기 (0) | 2024.06.07 |